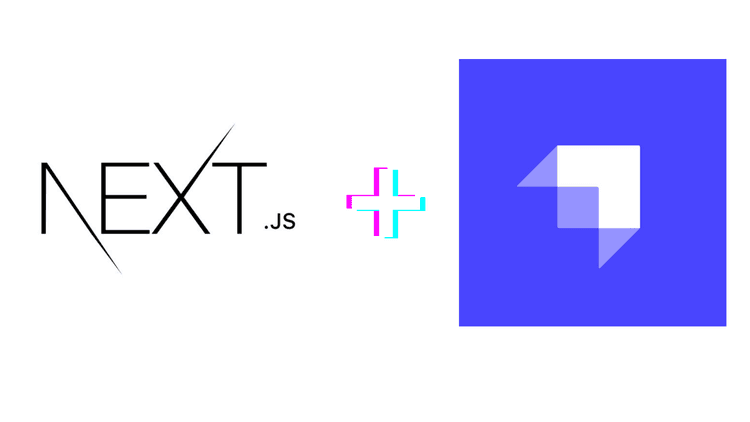
Creating a CMS-Powered Blog with Next.js and Strapi
August 20, 2023
In today's digital landscape, having a dynamic and content-rich blog is essential for engaging your audience and driving traffic to your website. Content Management Systems (CMS) and modern web development frameworks have made it easier than ever to create and manage such blogs. In this tutorial, we'll explore the process of building a CMS-powered blog using two powerful tools: Next.js and Strapi.
Why Next.js and Strapi?
Next.js is a popular React framework that enables server-side rendering, static site generation, and client-side rendering. It provides a seamless user experience by improving page load times and optimizing SEO. Strapi, on the other hand, is a headless CMS that offers a flexible content management system, allowing you to create, manage, and organize your blog content with ease.
Prerequisites
Before we begin, make sure you have the following tools installed:
- Node.js and npm: Make sure you have the latest versions of Node.js and npm installed on your system.
- Text Editor: Choose a code editor of your choice, such as Visual Studio Code or Sublime Text.
Step 1: Setting up the Strapi CMS
- Install Strapi globally using npm:
npm install -g strapi
- Create a new Strapi project:
strapi new my-blog
- Follow the prompts to configure your Strapi project. You can choose the "Blog" template to get started quickly.
- Define content types such as "Post" with fields like title, content, date, and author.
Step 2: Creating the Next.js Application
- Initialize a new Next.js project:
npx create-next-app my-blog-app
- Navigate to the project directory:
cd my-blog-app
- Install required dependencies:
npm install axios
Step 3: Fetching Data from Strapi
- In the pages/index.js file, import the axios library:
import axios from 'axios';
javascriptCopy codeimport axios from 'axios';
- Create a function to fetch blog posts from Strapi:
async function getBlogPosts() {
try {
const response = await axios.get('http://localhost:1337/posts');
return response.data;
} catch (error) {
console.error('Error fetching blog posts:', error);
return [];
}
}
- Use the getBlogPosts function to fetch and display blog posts on the index page.
Step 4: Displaying Blog Posts
- In the pages/index.js file, use the getBlogPosts function to fetch data:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
function Home() {
const [posts, setPosts] = useState([]);
useEffect(() => {
async function fetchPosts() {
const data = await getBlogPosts();
setPosts(data);
}
fetchPosts();
}, []);
return (
<div> <h1>Welcome to My Blog</h1> <ul> {posts.map(post => ( <li key={post.id}> <h2>{post.title}</h2> <p>{post.content}</p> </li> ))} </ul> </div>
);
}
export default Home;
Conclusion
Congratulations! You've successfully created a CMS-powered blog using Next.js and Strapi. This powerful combination allows you to build a fast and SEO-friendly blog while managing your content efficiently with Strapi's user-friendly interface. With the flexibility of Strapi and the performance benefits of Next.js, you're well-equipped to engage your audience with compelling blog content. Happy blogging!