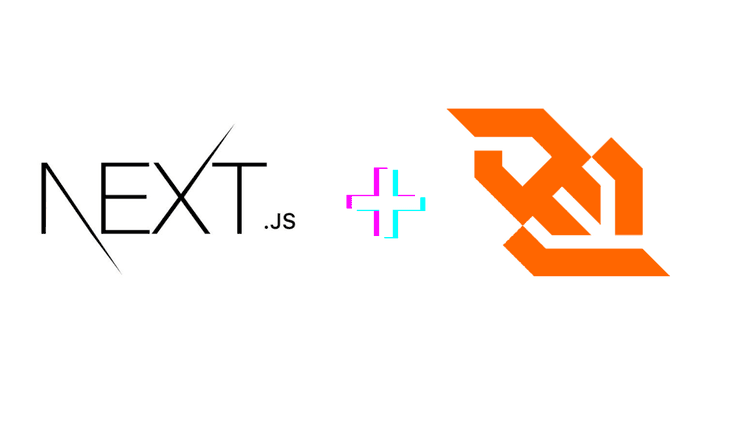
Real-Time Notifications in Next.js with WebSockets
August 20, 2023
In the fast-paced digital world, real-time notifications are a must-have feature for engaging user experiences. Whether you're building a messaging app, a social network, or an e-commerce platform, providing users with instant updates is essential. In this tutorial, we'll explore how to implement real-time notifications in a Next.js application using WebSockets. Buckle up for a journey into the world of seamless and interactive notifications!
Prerequisites
- Basic understanding of Next.js and React
- Familiarity with JavaScript and server-side programming
Understanding WebSockets
WebSockets offer a two-way communication channel between the client (browser) and the server, allowing for real-time data exchange without the overhead of HTTP requests. This makes them ideal for implementing features like real-time notifications.
Setting Up the Project
- Initialize Next.js Project: Create a new Next.js project using npx create-next-app and navigate into the project directory.
- Install Dependencies: Install the necessary packages, including socket.io-client for the client-side WebSocket implementation and socket.io for the server-side implementation.
Implementing Real-Time Notifications
Server-Side
- Set Up WebSocket Server: In a new file, such as websocketServer.js, create a WebSocket server using the socket.io library. Listen for connections and emit events to clients.
- Emit Notifications: When an event occurs that triggers a notification (e.g., a new message), emit that event to the appropriate clients.
Client-Side
- Connect to WebSocket Server: In a component, such as NotificationContainer.js, import socket.io-client and establish a connection to the WebSocket server.
- Listen for Notifications: Set up listeners for the events you're emitting from the server. When a notification event is received, update the UI to display the notification to the user.
Enhancing the User Experience
- Displaying Notifications: Use a UI component, such as a toast or a notification badge, to display real-time notifications to users. You can use libraries like react-toastify for a sleek notification display.
- Handling User Interaction: Allow users to interact with notifications, such as marking them as read or clicking to navigate to relevant sections of your app.
Testing the Real-Time Feature
- Open Multiple Tabs: Open your Next.js app in multiple browser tabs or devices to simulate different users.
- Trigger Notifications: From one tab, trigger events that should generate notifications. Observe how other tabs receive and display these notifications in real time.
Code Example (Client-Side):
// NotificationContainer.js
import React, { useEffect } from 'react';
import io from 'socket.io-client';
import { toast } from 'react-toastify';
const NotificationContainer = () => {
useEffect(() => {
const socket = io.connect('http://localhost:5000'); // Replace with your server's URL
socket.on('notification', (message) => {
toast.info(`New Notification: ${message}`);
});
return () => {
socket.disconnect();
};
}, []);
return (
<div>
{/* Your UI and Components */}
</div>
);
};
export default NotificationContainer;
Conclusion
Implementing real-time notifications in your Next.js app using WebSockets adds a layer of interactivity and engagement that modern users expect. The power of instant updates transforms your app from static to dynamic, providing users with a seamless and immersive experience.
WebSockets enable you to bridge the gap between the client and the server, facilitating real-time communication that can be harnessed for various features beyond notifications. By mastering WebSockets, you're equipping yourself with a valuable tool for building modern, responsive, and interactive web applications.