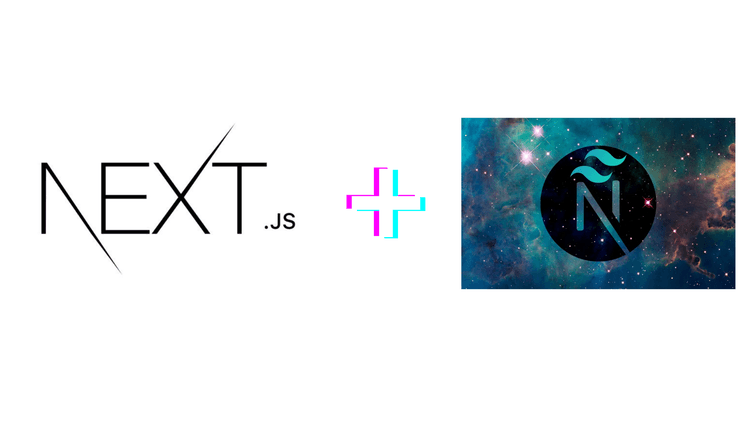
How to integrate nextjs in next-theme in page route
September 3, 2023
Integrating Next.js with the `next-themes` library allows you to easily manage theme switching in your Next.js application. Here's a step-by-step guide with more detailed explanations:
**1. Create a Next.js Project:**
If you haven't already, set up a Next.js project using the following command:
npx create-next-app my-nextjs-app
**2. Install `next-themes`:**
Inside your Next.js project folder, install the `next-themes` package:
npm install next-themes
# or
yarn add next-themes
**3. Configure the `next-themes` Provider:**
In your Next.js app, you need to configure the `next-themes` provider. This is typically done in your custom `_app.js` file (located in the `pages` directory).
// pages/_app.js
import { ThemeProvider } from 'next-themes';
function MyApp({ Component, pageProps }) {
return (
<ThemeProvider defaultTheme="light">
{/* Your app content */}
<Component {...pageProps} />
</ThemeProvider>
);
}
export default MyApp;
In the code above:
- We import the `ThemeProvider` from `next-themes`.
- We wrap our app with the `ThemeProvider` component.
- We set the `defaultTheme` prop to specify the initial theme (e.g., 'light' or 'dark').
**4. Create a Theme Toggle Button (Optional):**
To allow users to switch between themes, you can create a theme toggle button. Here's a basic example:
// components/ThemeToggle.js
import { useTheme } from 'next-themes';
function ThemeToggle() {
const { theme, setTheme } = useTheme();
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light');
};
return (
<button onClick={toggleTheme}>
Toggle Theme ({theme === 'light' ? 'Dark' : 'Light'})
</button>
);
}
export default ThemeToggle;
**5. Use the ThemeToggle Component:**
You can then use the `ThemeToggle` component wherever you want in your app to allow users to switch themes.
**6. Style Your Themes:**
To style your themes, you can create separate CSS or SCSS files for each theme and use CSS variables or dynamic styles to apply the theme-specific styles.
**7. Configure Additional Themes (Optional):**
By default, `next-themes` supports 'light' and 'dark' themes. If you want to add more themes or customize the themes further, you can do so by configuring the `themes` prop in the `ThemeProvider`.
**8. Testing and Customization:**
Test your theme switching functionality thoroughly, and customize the themes and styles to match your application's design.
**9. Documentation:**
Document how users can switch themes and provide clear instructions on using the theme toggle button if you've added one.
**10. Publish Your Next.js App:**
Finally, publish your Next.js app with integrated theme switching to make it accessible to users.
By following these steps, you can effectively integrate `next-themes` into your Next.js application, enabling theme switching for your users. The `next-themes` library simplifies the process of managing themes, making it easier to provide a seamless user experience with different visual styles.