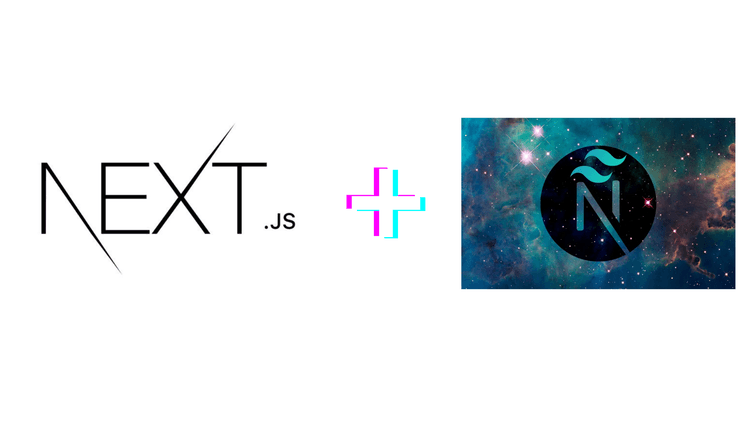
How to integrate nextjs in next-theme in approute
September 3, 2023
To integrate route-based theming using next-themes in your Next.js app, you can follow these steps:
- Set Up next-themes :First, make sure you have the next-themes and next/router packages installed in your Next.js project if you haven't already. You can install them using npm or yarn:
npm install next-themes
# or
yarn add next-themes
- Create Theme Provider and Context:Create a ThemeProvider and a context for handling theme changes. You can do this in a new file, such as theme-provider.tsx:In this code:
// theme-provider.tsx
"use client"
import * as React from "react"
import { ThemeProvider as NextThemesProvider } from "next-themes"
import { type ThemeProviderProps } from "next-themes/dist/types"
export function ThemeProvider({ children, ...props }: ThemeProviderProps) {
return <NextThemesProvider {...props}>{children}</NextThemesProvider>
}
- We create a ThemeProvider component that wraps around NextThemeProvider from next-themes.
- We manage the theme state using the useState hook.
- We provide a useTheme hook to access the theme context.
- Integrate Theme Provider in Your App:In your Next.js app, integrate the ThemeProvider you created in step 2. Typically, this is done in your layout.tsx file:
// app/layout.tsx
<html lang="en" suppressHydrationWarning>
<body className={inter.className}>
<ThemeProvider attribute="class" defaultTheme="system" enableSystem>
</ThemeProvider>
</body>
</html>
Create Theme Switcher Component :If you want to provide users with the option to switch themes, you can create a theme switcher component. This component allows users to toggle between light and dark themes or any other themes you configure.
mode-tooggle.tsx
"use client";
import * as React from "react";
import { FaMoon, FaSun } from "react-icons/fa";
import { useTheme } from "next-themes";
export function ModeToggle() {
const { theme, setTheme } = useTheme();
return (
<div>
{theme === "dark" ? (
<button onClick={() => setTheme("light")}>
<FaMoon className="w-6 h-6" />
</button>
) : (
<button onClick={() => setTheme("dark")}>
<FaSun className="w-6 h-6" />
</button>
)}
</div>
);
}
Create a header component in React for your Next.js app.
import React from "react";
import Link from "next/link";
import ModeToggle from "./mode-toggle"; // Assuming ModeToggle is a valid component
export default function Header() {
return (
<div className="flex flex-row justify-between mx-2 max-w-6xl sm:mx-auto items-center py-6">
<div className="flex flex-row">
<Link href="/">Home</Link>
</div>
<div className="flex flex-row mx-2 items-center">
<ModeToggle />
<h2>App Name</h2> {/* Corrected the closing </h2> tag */}
</div>
</div>
);
}
- Styling and Customization:Ensure that you have styles for different themes and customize them as needed. You can use CSS variables or dynamic styling based on the current theme.
- Testing and Documentation:Test your route-based theming functionality thoroughly, and document how routes affect theme changes in your application.
- Publish Your Next.js App:Finally, publish your Next.js app with app route-based theming to make it accessible to users.
By following these steps, you can integrate route-based theming using next-themes in your Next.js app. This allows you to switch themes based on routes or specific conditions, providing a dynamic and personalized user experience.